- Multithreaded Prime Number Generator
This is more of an intermediate topic. If you're just getting started on the .NET Compact Framework 2.0, you can read this to install the tools and this to write your first app.
In this tutorial, you learn how to write a multithreaded program that does some background processing and yet maintains a UI that's responsive to the user.
A multithreaded program is useful in that it gives the user a responsive UI and at the same time be productive in some background work, such as doing long calculations and IO. How do you decide when to start a thread? If the program is about to be engaged in some long processing, and you do not want to user to wait, then it's better to start a thread that works on the processing, while leaving the main UI thread running. This is known as threading.
As usual, we will illustrate how to do threading with this simple step-by-step tutorial. We will write a program that keeps calculating prime numbers in the background, while leaving the UI responsive.
Categories: [Threading_] Tags: [netcf2] [Compact Framework] [Windows Mobile] [Threading]
In this tutorial, you learn how to write a multithreaded program that does some background processing and yet maintains a UI that's responsive to the user.
A multithreaded program is useful in that it gives the user a responsive UI and at the same time be productive in some background work, such as doing long calculations and IO. How do you decide when to start a thread? If the program is about to be engaged in some long processing, and you do not want to user to wait, then it's better to start a thread that works on the processing, while leaving the main UI thread running. This is known as threading.
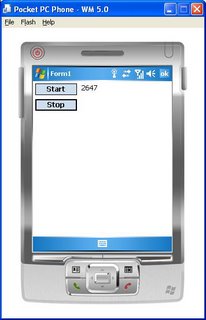
- Create a C# Windows Mobile 5.0 PPC Device Application project in Visual Studio .NET 2005.
- Change the form's MinimizeBox property to False (so that you can easily close it by clicking the OK at the top-right corner).
- Add 2 Button controls and a Label control. Call them btnStart, btnStop and label1. Set their Text properties to: "Start", "Stop" and [empty string] respectively.
- Go to the code view (press F7). Add the line "using System.Threading;" to the top, so that your code can use the Threading namespace.
- Add 2 private attributes to your partial class: private string textToShow, private bool runThread.
- Go back to design view (press Shift-F7). Double-click on the Start button and enter the following code to start a thread.
- In design view, double-click the Stop button and give it this code which informs the thread to stop:
- Back in design view, select the Form, and in the Properties panel, click on the Events button (the one with the thunderbolt image on it). Double-click on the Closing event. We will write one line of code for the closing event of the form, also to end the thread:
- Finally, add these 2 methods to the partial class:
- Compile, deploy to emulator and run. Test it by clicking the buttons. You will see that the prime numbers are being calculated and displayed, while leaving UI not blocked (i.e. still responsive).
- Notice that in the code, the label can't be updated directly from the worker thread. This is because the label object belongs to the main process thread and has to be synchronously updated through an Invoke call.
Thread t = new Thread(new ThreadStart(WorkerThread));
runThread = true;
t.Start();
runThread = false;
runThread = false;
private void WorkerThread()
{
int n = 3;
while (runThread)
{
bool isPrime = true;
for (int f = 3; f <= Math.Sqrt(n); f += 2)
{
if (n % f == 0)
{
isPrime = false;
break;
}
}
if (isPrime)
{
textToShow = n.ToString();
this.Invoke(new EventHandler(Update_label));
}
n += 2;
}
}
private void Update_label(object sender, EventArgs e)
{
label1.Text = textToShow;
}
Categories: [Threading_] Tags: [netcf2] [Compact Framework] [Windows Mobile] [Threading]
8 Comments:
Nice article, thanks a lot :)
This will make my Mobile apps more responsive !
Cheers
Laurent
Very nice article and a simple to-the-point example! Thanks much for getting me out of a jam!
Thank you very much for the sample :)
Hi Eric and Tom,
Thank you for your kind words.
-daniel
Great Blog and Nice Tips
Thanks for this share,GBU!
Droping by...
婚活
オフィス家具
婚活
お見合いパーティー
債務整理
過払い
Post a Comment
<< Home